First steps
[Top] [Next]
The first goal is to simply drawing a grid. More specifically can we vary the shape of the grid tiles in a meaningful way like in renowned explorers or Townscaper, or this science paper, and a nice demo.
Even smaller for the first step we’ll just draw a simple mesh at runtime in Unity.
To create and draw a mesh at runtime in Unity one has to:
- create an empty GameObject
- add a MeshFilter
- add MeshRenderer
- implement a Mesh generation object
To make sure the mesh is indeed as expected, a rotation behavior is added to make the object rotate every second.
To define a mesh, a MeshDefinition
class is added which simply looks like this:
[Serializable]
public class MeshDefinition
{
public Vector3[] vertices;
public Vector2[] uv;
public int[] triangles;
public bool IsValid() => vertices.Length >= 3 && triangles.Length >= 3 && uv.Length >= 3;
}
Although subject to change, the code the core of the mesh generation is following call in MeshGenerator
:
private void UpdateMeshDefinition(Mesh mesh, MeshDefinition definition)
{
mesh.vertices = definition.vertices;
mesh.uv = definition.uv;
mesh.triangles = definition.triangles;
mesh.RecalculateNormals();
mesh.RecalculateBounds();
mesh.RecalculateTangents();
}
The results should show up as below:
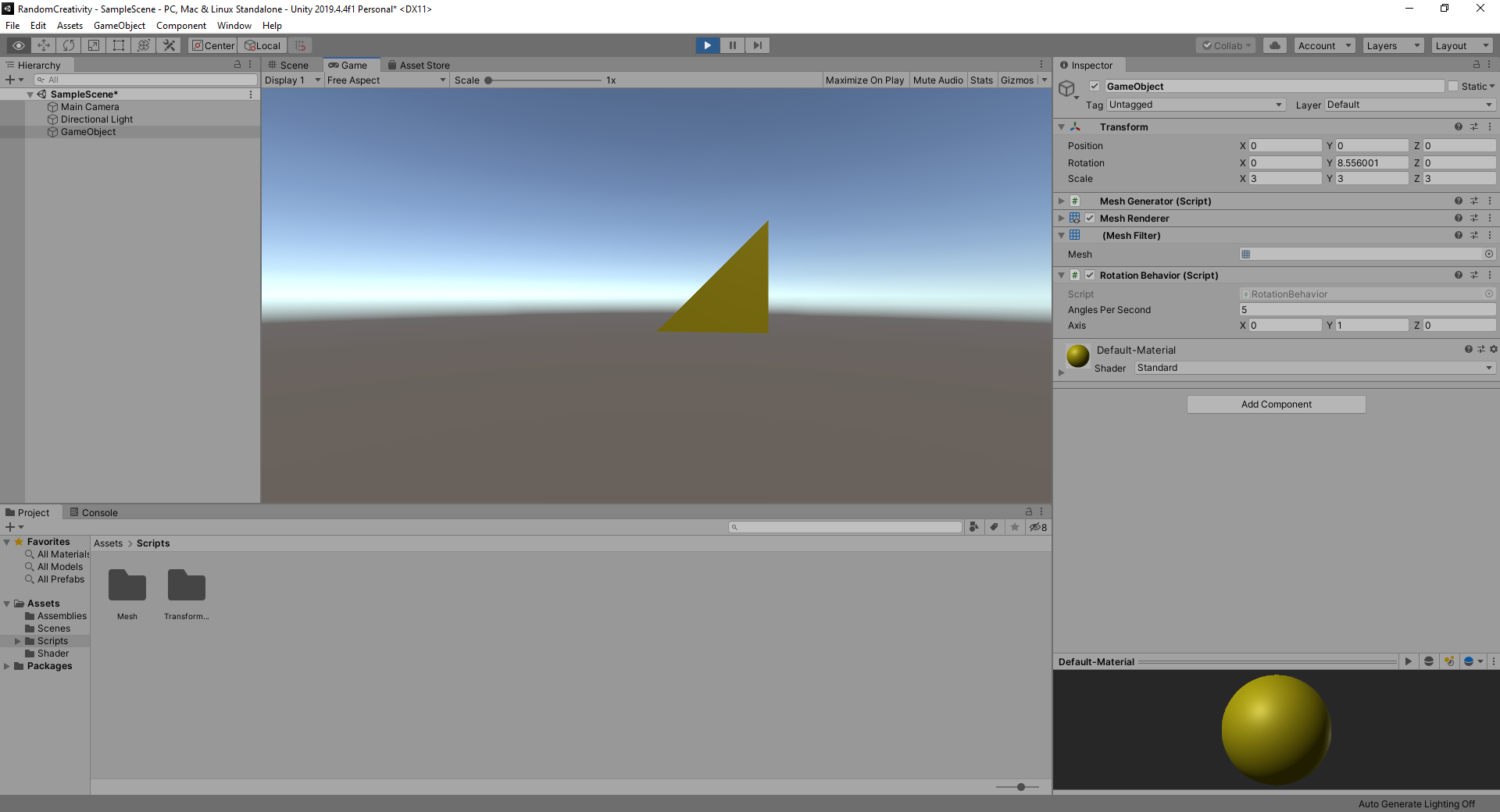
Scope Creep (next step considerations)
There are a couple of shortcomings with this approach, which could be added:
- No editor interactivity, you can’t see the mesh as you’re changing it in the editor
- The center of the mesh is defined by the definition. I’m not sure if that’s ok or if an anchor point should be defined… I’m inclined to say the former, ie it’s up to the user to fix the input data, BUT it would be nice and more user friendly if this was adjusted for the user. For now there’s no intention to address this.
- Meshes are not doublesided. Same arguments as in the previous point apply.